Swordplay
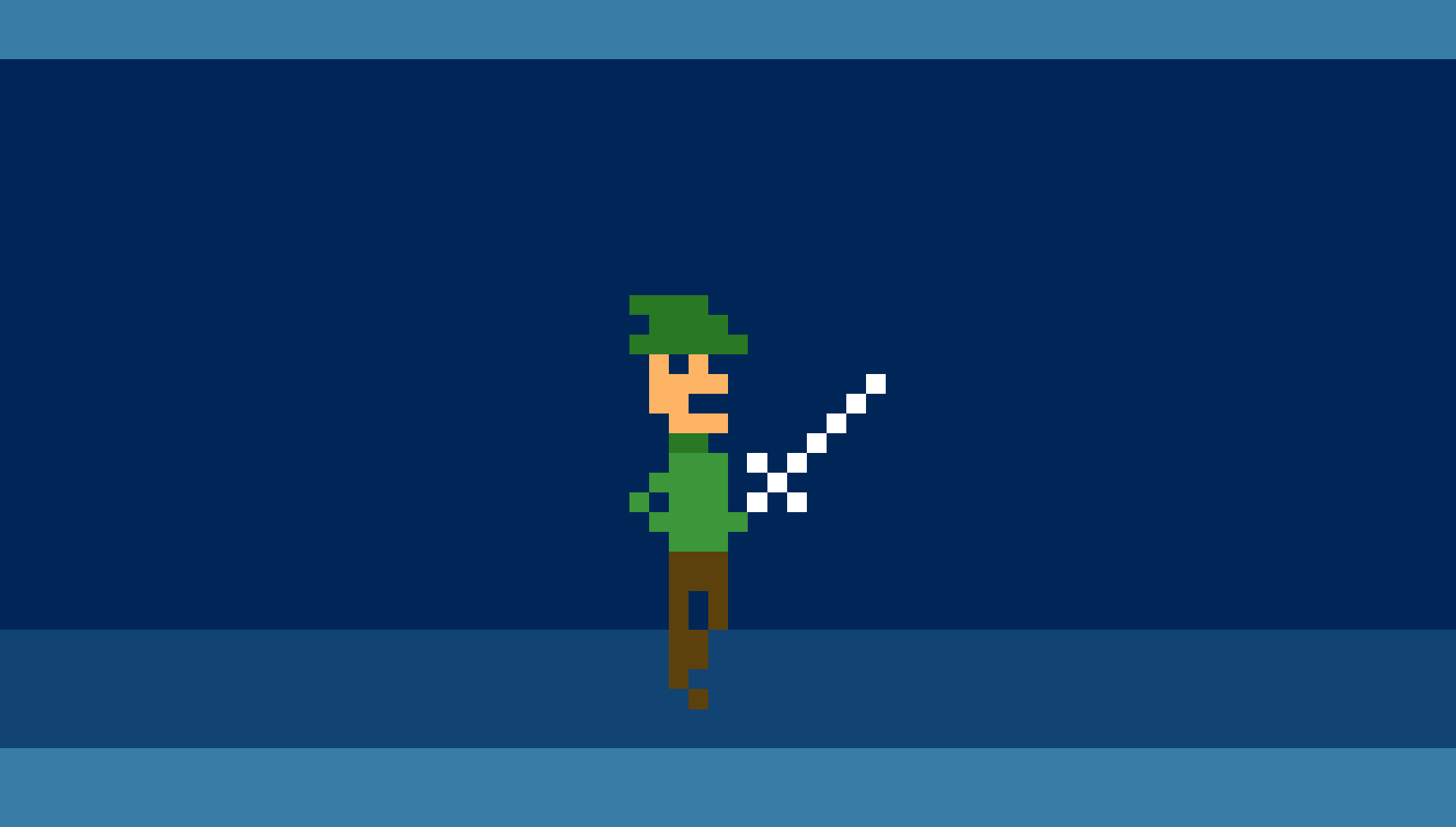
Now, we’re not going to be able to get very far if we can’t attack the enemies. So I need a way to attack.
When I was designing the game in my head, one of the Atari’s limitations forced me to make a choice: the joystick controller for the 2600 has one button. Which means I have to pick one answer: am I designing a game where I can jump, swing a sword, or shoot a missile?
I was already inspired by the likes of Pitfall, but I imagined a little rogue having to enter and climb a tower full of monsters. Attacking them with a sword felt more like the kind of game I had in my head. (Also, I didn’t feel like also figuring how to calculate an arc for his trajectory in assembly…)
Now, comes the tricky part.
Sprites in the Atari are stored as a single byte of data, and you (typically) get to draw them once per scanline. Each bit gives you 1 pixel. Since my guy is already 8 pixels wide, I can’t really fit a sword by just adding some extra animation frames with a sword in it. I could revisit the multi-sprite technique I used for the score, but I don’t want to mess with my kernel any more, and I also want the sword to be a different colour — I don’t think being the same colour as his face and clothes will look right…
So, I need to use another trick to display multiple sprites: flickering. This doesn’t look so good on our modern displays, but it exploited the persistant image on a CRT television. The old PAL and NTSC television image formats would encode an image 50 and 60 times per second, respectively. The TV would interlace these together on alternating lines, allowing the ghosting in the CRT phosphors to give the illusion of a persistent image, ultimately giving video of 25 or 30 frames across both fields. We can make use of this to display two different sprites at once: alternating quickly between the first and second images. We don’t get the interlacing of PAL/NTSC, so the images become transparent, and you will still notice the flickering, but this tecnique was employed a lot on the Atari.
I now have two extra states: Attacking, and RenderingAttack.
When I press the button on the controller, we set Attacking to true
.
While Attacking is true
, we toggle RenderingAttack with every gameloop, otherwise we set it to false
.
When RenderingAttack is false
, we render our player as normal. When it is true
, we instead render the sword.
Becasue we don’t want the sword to be in the middle of his torso, we have to offset it left or right depending on which way he’s facing. So we also have to swap out the player X position, offset it for the sword, and swap it back in when we’re back to the player.
Put that together and we get this:
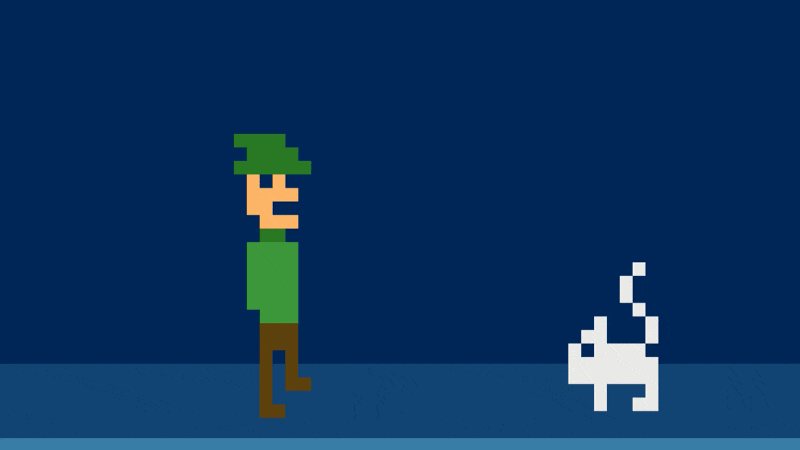
I’ve edited this GIF so that it dims the player sprite instead of flickering it. Recording the emulator to a gif and sticking it on an HTML page makes the flicker a bit too pronounced.
Neat. Took a bit of faffing with the animation and positioning to get it to work, but I think it’s quite effective. The player is animated on fours (each frame of animation is displayed for four frames on the television.). Because of the alternating images, the sword takes twice as long to animate, so I’m animating it on twos.
Now, to make the sword have an effect.
As I mentioned before, the Atari makes it easy to check if things have collided. All I need to do is check whether P0 (the player) and P1 (an item or emeny) sprited have collided, and I can take action.
The Atari has a single flag for when the P0 and P1 sprites collide, but I need to know if they collided with the player, or the sword. This is made easy by just looking at whether I’ve just rendered the sword or the player. I check the collisions after the image is rendered, but before the next frame is prepared or the input is checked, so I know I can trust the collision is between whatever I’ve just finished drawing.
If the RenderingAttack is true
, then the enemy hit the sword; kill the enemy and stop drawing it. If it’s false
, then the enemy hit you; you lose a life.
Furthermore, when it collides, there’s the extra issue of determining whether you’ve hit the top floor or bottom floor entity — it only tell you that something collided. So I also have to check the Y-position of the player. Luckily there’s no position where both the top and bottom floor enemies could hit you.
The next steps will be differentiating between enemies and items (that money bag in all the screenshots shouldn’t kill you), as well as adding a bit of difficulty by making stronger enemies take multiple hits.
I’ve disabled autoplay on this video because of the flickering effect.
I’ll also have to think of what will happen when you lose a life, and what will happen when you lose all of them.